Prebuilt Checkout page
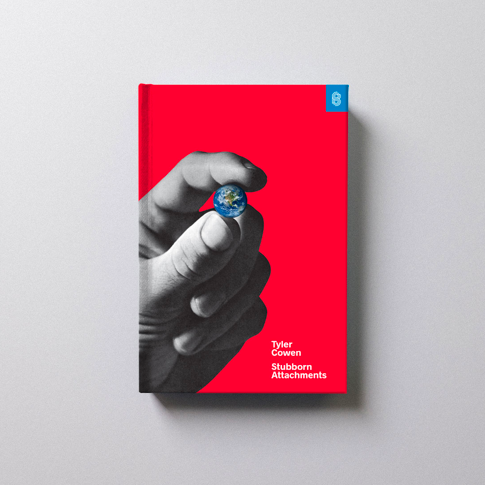
Stubborn Attachments
$20.00
Explore a full, working code sample of an integration with Stripe Checkout. The client- and server-side code redirects to a prebuilt payment page hosted on Stripe.
Before you get started, confirm the payment methods you want to offer in your payment method settings. We enable cards and other common payment methods for you by default, and we recommend that you enable additional payment methods that are relevant for your business and customers.
Set up the server
Install the Stripe Ruby library
Install the Stripe ruby gem and require it in your code. Alternatively, if you’re starting from scratch and need a Gemfile, download the project files using the link in the code editor.
Create a Checkout Session
Add an endpoint on your server that creates a Checkout Session. A Checkout Session controls what your customer sees on the payment page such as line items, the order amount and currency, and acceptable payment methods. We enable cards and other common payment methods for you by default, and you can enable or disable payment methods directly in the Stripe Dashboard.
Define a product to sell
Always keep sensitive information about your product inventory, such as price and availability, on your server to prevent customer manipulation from the client. Define product information when you create the Checkout Session using predefined price IDs or on the fly with price_data.
Choose the mode
Checkout has three modes: payment
, subscription
, or setup
. Use payment
mode for one-time purchases. Learn more about subscription and setup modes in the docs.
Supply success and cancel URLs
Specify URLs for success and cancel pages—make sure they’re publicly accessible so Stripe can redirect customers to them. You can also handle both the success and canceled states with the same URL.
Redirect to Checkout
After creating the session, redirect your customer to the URL for the Checkout page returned in the response.
Build your checkout
Add a success page
Create a success page for the URL you provided as the Checkout Session success_url to display order confirmation messaging or order details to your customer. Stripe redirects to this page after the customer successfully completes the checkout.
Add a canceled page
Add another page for cancel_url
. Stripe redirects to this page when the customer clicks the back button in Checkout.
Add an order preview page
Finally, add a page to show a preview of the customer’s order. Allow them to review or modify their order—as soon as they’re sent to the Checkout page, the order is final and they can’t modify it without creating a new Checkout Session.
Add a checkout button
Add a button to your order preview page. When your customer clicks this button, they’re redirected to the Stripe-hosted payment page.
Congratulations!
You have a basic Checkout integration working. Learn how to customize the appearance of your checkout page.
Next steps
Fulfillment
Set up a webhook to fulfill orders after a payment succeeds. Webhooks are the most reliable way to handle business-critical events.
Payouts
Learn how to move funds out of your Stripe account into your bank account.
Refunds
Handle requests for refunds by using the Stripe API or Dashboard.
Prebuilt Checkout page
Explore a full, working code sample of an integration with Stripe Checkout. The client- and server-side code redirects to a prebuilt payment page hosted on Stripe.
Before you get started, confirm the payment methods you want to offer in your payment method settings. We enable cards and other common payment methods for you by default, and we recommend that you enable additional payment methods that are relevant for your business and customers.
Set up the server
Install the Stripe Ruby library
Install the Stripe ruby gem and require it in your code. Alternatively, if you’re starting from scratch and need a Gemfile, download the project files using the link in the code editor.
Create a Checkout Session
Add an endpoint on your server that creates a Checkout Session. A Checkout Session controls what your customer sees on the payment page such as line items, the order amount and currency, and acceptable payment methods. We enable cards and other common payment methods for you by default, and you can enable or disable payment methods directly in the Stripe Dashboard.
Define a product to sell
Always keep sensitive information about your product inventory, such as price and availability, on your server to prevent customer manipulation from the client. Define product information when you create the Checkout Session using predefined price IDs or on the fly with price_data.
Choose the mode
Checkout has three modes: payment
, subscription
, or setup
. Use payment
mode for one-time purchases. Learn more about subscription and setup modes in the docs.
Supply success and cancel URLs
Specify URLs for success and cancel pages—make sure they’re publicly accessible so Stripe can redirect customers to them. You can also handle both the success and canceled states with the same URL.
Redirect to Checkout
After creating the session, redirect your customer to the URL for the Checkout page returned in the response.
Build your checkout
Add a success page
Create a success page for the URL you provided as the Checkout Session success_url to display order confirmation messaging or order details to your customer. Stripe redirects to this page after the customer successfully completes the checkout.
Add a canceled page
Add another page for cancel_url
. Stripe redirects to this page when the customer clicks the back button in Checkout.
Add an order preview page
Finally, add a page to show a preview of the customer’s order. Allow them to review or modify their order—as soon as they’re sent to the Checkout page, the order is final and they can’t modify it without creating a new Checkout Session.
Add a checkout button
Add a button to your order preview page. When your customer clicks this button, they’re redirected to the Stripe-hosted payment page.
Congratulations!
You have a basic Checkout integration working. Learn how to customize the appearance of your checkout page.
Next steps
Fulfillment
Set up a webhook to fulfill orders after a payment succeeds. Webhooks are the most reliable way to handle business-critical events.
Payouts
Learn how to move funds out of your Stripe account into your bank account.
Refunds
Handle requests for refunds by using the Stripe API or Dashboard.
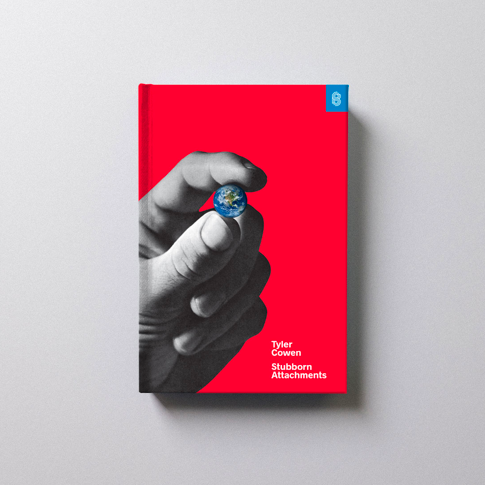